Online Manual: Plugins
Toolbar | Orders | Reports | Formulae | Techniques | Components | Profiles
Updates | Configuration | Customization | Hotkeys | Plugins | FAQ
Note: This page is work in progress and will change often in the next couple of weeks.
1. Plugins
This feature allows third party applications to collect and process orders, reports and profiles. For example a guild website can allow their members to upload their orders via HTTP or FTP.
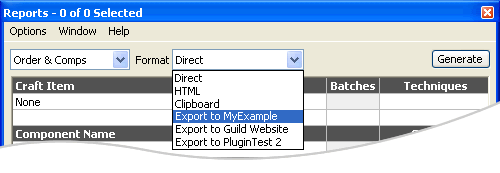
If a plugin is found at progam launch, HCC will add a new entry to the format dropdown at the reports screen: Export to {Plugin Name}.
Once you press "Generate", HCC will generate several files into the Temp\ folder and Plugins\{Plugin Name}\export.bat will be launched in minimized state with seven parameters:
export.bat {path_to_exported_files} {hcc-version} {database-date} {order|bonus} [name] [shard] [email]
Whether or not the dos-window is closed on completion is only up to the batch file (remove "pause"-lines). HCC does not monitor errors the .bat might cause. It is recommended to use pause for errors.
order.xml
The actual HCC order. If you offer this file as download the crafter can load it with HCC and make changes to the file.
profile.xml
Profile of the user (will only be created if the user selected a profile). If you offer this file as download a user can load it with HCC and make changes to the file.
report.html, report.xml, report.xml
The reports of an order contain pre-rendered data to be used to display by third party applications who don't have access to the HCC XML database. This format cannot be imported back into HCC.
2. Security tips for users
Important Notice: Never install a plugin from an untrusted source, only obtain them from people you know and trust.
Plugin support requires Windows 2000, XP, Vista, 7 or newer. Some plugins might depend on a specific Windows version or other software requirements. Please review the plugin readme for detailed information.
When you install a plugin make sure you extract it to the right place and maintain the provided directory structure inside the zip.
The correct directory tree is:
3. Plugin Development
To get started take a look at "HCC Online Orders" example inside the Plugins\ folder. Duplicate the folder with your own plugin name, customize export.bat and select "Export to {your plugin}" at the Reports Screen inside HCC.
Parameters:
All parameters are enclosed with quotes, use %~ to access the actual contents
%~1 = Path to exported files (%1 is with quotes, no trailing backslash)
%~2 = Type [order|bonus] (%2 is with quotes)
%~3 = Version of HCC (e.g. 2.30, %3 is with quotes)
%~4 = Database of HCC (e.g. 2009-03-16, %4 is with quotes)
%~5 = Player name (can be empty, %3 is with quotes)
%~6 = Shard name [chaos|order|blight] (can be empty, %4 is with quotes)
%~7 = Email address (can be empty - even if player/shard are set, %5 is with quotes)
Empty Plugin:
Example 1: Upload via FTP
The following example will upload "%~1\report.html" ("c:\Program Files\HCC2\Temp\report.html") to test/123456789.html on www.example.com via FTP. Please note that even anonymous FTP server demand a password (just use any string you like, sometimes an email is required). & needs to be escaped as ^& outside quotes.
WARNING: If you offer FTP uploads never let the .bat file upload into your executable webroot, reachable for everyone: The username and password are exposed in the batch file, everyone with an FTP Client can upload any file they want, even .php files with contents that could wipe your server HDD with the blink of an eye. Always upload into a directory that cannot be reached from the web!
Example 2: Upload via HTTP POST
This will upload %~1\order.xml (c:\Program Files\HCC2\Temp\order.xml) as well as two reports and the profile to submit.php on www.example.com via HTTP POST. By default "curl" isn't part of Windows and must be provided with your plugin. Please note that there are no additional quotes needed inside -F "". This example uses %curl_profile% from the empty plugin snipped above to also include the profile, if provided.
WARNING: Never move uploaded files into your executable webroot, reachable for everyone: Anyone can change the export.bat and upload any file they want, even .php files with contents that could wipe your server HDD with the blink of an eye. Always upload into a directory that cannot be reached from the web!
Example 3: Printing
This will send "%~1\report.txt" ("c:\Program Files\HCC2\Temp\report.txt") to your default printer.
Example 4: Only allow a specific export type
Processing:
WARNING: Directly displaying any of the user uploads is a very bad idea!
Always upload into a directory that cannot be reached from the web! Write a small parser script that loads the files for your users and checks the contents before initiating a transfer. The safest choice is to process the .xml files! HTML and TXT are the unsafest options. It is your obligation to review and check the file the user uploaded to your server. TrID (http://mark0.net/soft-trid-e.html) is an excellent app that can help detect actual filetypes, regardless of their mimetype or extension.
The safest method is to process only the XML files by using any mature XML-Parser class of your programming language of choice. Always replace html-entities if you output contents from any upload! People can other embed javascripts to steal users cookies off your website, or other XSS attacks.
To process HTML orders it's recommend to load the HTML file and strip all tags except h1, table, tr, th and td. Of course you can also strip off the styles, use your own colors or link directly to the official stylesheet.
4. Distribution
Distribute your plugin by putting a zipped version on a Website and send the link via email or post it in a forum post, maybe with some instructions. Don't send the plain batch file directly via email as attachment. All major mail servers filter out executable files, even if they are zipped. Please provide a small readme.txt with your plugin, containing system requirements, known issues and a way to contact you.
Please make sure you include the Plugin Folder into the zip! If you use WinZip or 7Zip the correct method is to rightclick on "MyUploader" and select Zip -> Add to "MyUploader.zip".
Top
Updates | Configuration | Customization | Hotkeys | Plugins | FAQ
Note: This page is work in progress and will change often in the next couple of weeks.
1. Plugins
This feature allows third party applications to collect and process orders, reports and profiles. For example a guild website can allow their members to upload their orders via HTTP or FTP.
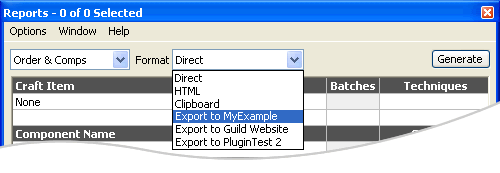
If a plugin is found at progam launch, HCC will add a new entry to the format dropdown at the reports screen: Export to {Plugin Name}.
Once you press "Generate", HCC will generate several files into the Temp\ folder and Plugins\{Plugin Name}\export.bat will be launched in minimized state with seven parameters:
export.bat {path_to_exported_files} {hcc-version} {database-date} {order|bonus} [name] [shard] [email]
Whether or not the dos-window is closed on completion is only up to the batch file (remove "pause"-lines). HCC does not monitor errors the .bat might cause. It is recommended to use pause for errors.
order.xml
The actual HCC order. If you offer this file as download the crafter can load it with HCC and make changes to the file.
profile.xml
Profile of the user (will only be created if the user selected a profile). If you offer this file as download a user can load it with HCC and make changes to the file.
report.html, report.xml, report.xml
The reports of an order contain pre-rendered data to be used to display by third party applications who don't have access to the HCC XML database. This format cannot be imported back into HCC.
2. Security tips for users
Important Notice: Never install a plugin from an untrusted source, only obtain them from people you know and trust.
Plugin support requires Windows 2000, XP, Vista, 7 or newer. Some plugins might depend on a specific Windows version or other software requirements. Please review the plugin readme for detailed information.
When you install a plugin make sure you extract it to the right place and maintain the provided directory structure inside the zip.
The correct directory tree is:
CODE
HCC Installation Folder\
+ Plugins\
+ Example\
export.bat
readme.txt
+ MyUploader\
config.ini
export.bat
readme.txt
upload.exe
+ Images\
logo.bmp
hello_world.png
+ Yet Another Plugin\
export.bat
readme.txt
upload.exe
+ Plugins\
+ Example\
export.bat
readme.txt
+ MyUploader\
config.ini
export.bat
readme.txt
upload.exe
+ Images\
logo.bmp
hello_world.png
+ Yet Another Plugin\
export.bat
readme.txt
upload.exe
3. Plugin Development
To get started take a look at "HCC Online Orders" example inside the Plugins\ folder. Duplicate the folder with your own plugin name, customize export.bat and select "Export to {your plugin}" at the Reports Screen inside HCC.
Parameters:
All parameters are enclosed with quotes, use %~ to access the actual contents
%~1 = Path to exported files (%1 is with quotes, no trailing backslash)
%~2 = Type [order|bonus] (%2 is with quotes)
%~3 = Version of HCC (e.g. 2.30, %3 is with quotes)
%~4 = Database of HCC (e.g. 2009-03-16, %4 is with quotes)
%~5 = Player name (can be empty, %3 is with quotes)
%~6 = Shard name [chaos|order|blight] (can be empty, %4 is with quotes)
%~7 = Email address (can be empty - even if player/shard are set, %5 is with quotes)
Empty Plugin:
CODE
@ECHO OFF
IF (%1)==() GOTO ERRORHCC
IF (%2)==() GOTO ERRORHCC
IF (%3)==() GOTO ERRORHCC
IF (%4)==() GOTO ERRORHCC
IF NOT %~2==order GOTO ERRORBONUS
IF NOT EXIST "%~1\report.txt" GOTO ERROR
IF NOT EXIST "%~1\report.html" GOTO ERROR
IF NOT EXIST "%~1\report.xml" GOTO ERROR
IF NOT EXIST "%~1\order.xml" GOTO ERROR
IF NOT EXIST "%~1\profile.xml" GOTO PLUGINSTART
:PLUGINSTART
REM your code here
exit
:ERRORBONUS
echo Error - This plugin cannot process Bonus reports.
pause
exit
:ERRORHCC
echo Error - This file can only be launched through HCC.
pause
exit
:ERROR
echo Error - An unexpected issue occured. Please contact plugin developer or HCC support.
pause
exit
IF (%1)==() GOTO ERRORHCC
IF (%2)==() GOTO ERRORHCC
IF (%3)==() GOTO ERRORHCC
IF (%4)==() GOTO ERRORHCC
IF NOT %~2==order GOTO ERRORBONUS
IF NOT EXIST "%~1\report.txt" GOTO ERROR
IF NOT EXIST "%~1\report.html" GOTO ERROR
IF NOT EXIST "%~1\report.xml" GOTO ERROR
IF NOT EXIST "%~1\order.xml" GOTO ERROR
IF NOT EXIST "%~1\profile.xml" GOTO PLUGINSTART
:PLUGINSTART
REM your code here
exit
:ERRORBONUS
echo Error - This plugin cannot process Bonus reports.
pause
exit
:ERRORHCC
echo Error - This file can only be launched through HCC.
pause
exit
:ERROR
echo Error - An unexpected issue occured. Please contact plugin developer or HCC support.
pause
exit
Example 1: Upload via FTP
The following example will upload "%~1\report.html" ("c:\Program Files\HCC2\Temp\report.html") to test/123456789.html on www.example.com via FTP. Please note that even anonymous FTP server demand a password (just use any string you like, sometimes an email is required). & needs to be escaped as ^& outside quotes.
CODE
SET uid=%random%%random%%random%
SET script="%~1\ftpScript.txt"
DEL /Q %script%
ECHO open www.example.com>> %script%
ECHO user>> %script%
ECHO anonymous>> %script%
ECHO anon@example.com>> %script%
ECHO put "%~1\report.html" test/%uid%.html>> %script%
ECHO disconnect>> %script%
ECHO bye>> %script%
ftp -i -n -s:%script%
DEL /Q %script%
start http://www.example.com/process.php?uid=%uid%^&type=%2
pause
SET script="%~1\ftpScript.txt"
DEL /Q %script%
ECHO open www.example.com>> %script%
ECHO user>> %script%
ECHO anonymous>> %script%
ECHO anon@example.com>> %script%
ECHO put "%~1\report.html" test/%uid%.html>> %script%
ECHO disconnect>> %script%
ECHO bye>> %script%
ftp -i -n -s:%script%
DEL /Q %script%
start http://www.example.com/process.php?uid=%uid%^&type=%2
pause
WARNING: If you offer FTP uploads never let the .bat file upload into your executable webroot, reachable for everyone: The username and password are exposed in the batch file, everyone with an FTP Client can upload any file they want, even .php files with contents that could wipe your server HDD with the blink of an eye. Always upload into a directory that cannot be reached from the web!
Example 2: Upload via HTTP POST
This will upload %~1\order.xml (c:\Program Files\HCC2\Temp\order.xml) as well as two reports and the profile to submit.php on www.example.com via HTTP POST. By default "curl" isn't part of Windows and must be provided with your plugin. Please note that there are no additional quotes needed inside -F "". This example uses %curl_profile% from the empty plugin snipped above to also include the profile, if provided.
CODE
SET uid=%random%%random%%random%
curl.exe -F "order=@%~1\order.xml" -F "report=@%~1\report.xml" -F "report_html=@%~1\report.html" %curl_profile% -F "type=%~2" -F "hcc-version=%~3" -F "hcc-database=%~4" -F "name=%~5" -F "shard=%~6" -F "email=%~7" -F "uid=%uid%" http://www.example.com/submit.php
start http://www.example.com/process.php?uid=%uid%^&type=%2
curl.exe -F "order=@%~1\order.xml" -F "report=@%~1\report.xml" -F "report_html=@%~1\report.html" %curl_profile% -F "type=%~2" -F "hcc-version=%~3" -F "hcc-database=%~4" -F "name=%~5" -F "shard=%~6" -F "email=%~7" -F "uid=%uid%" http://www.example.com/submit.php
start http://www.example.com/process.php?uid=%uid%^&type=%2
WARNING: Never move uploaded files into your executable webroot, reachable for everyone: Anyone can change the export.bat and upload any file they want, even .php files with contents that could wipe your server HDD with the blink of an eye. Always upload into a directory that cannot be reached from the web!
Example 3: Printing
This will send "%~1\report.txt" ("c:\Program Files\HCC2\Temp\report.txt") to your default printer.
CODE
notepad /p "%~1\report.txt"
Example 4: Only allow a specific export type
CODE
IF NOT %~2==order GOTO ERROR
Processing:
WARNING: Directly displaying any of the user uploads is a very bad idea!
Always upload into a directory that cannot be reached from the web! Write a small parser script that loads the files for your users and checks the contents before initiating a transfer. The safest choice is to process the .xml files! HTML and TXT are the unsafest options. It is your obligation to review and check the file the user uploaded to your server. TrID (http://mark0.net/soft-trid-e.html) is an excellent app that can help detect actual filetypes, regardless of their mimetype or extension.
The safest method is to process only the XML files by using any mature XML-Parser class of your programming language of choice. Always replace html-entities if you output contents from any upload! People can other embed javascripts to steal users cookies off your website, or other XSS attacks.
To process HTML orders it's recommend to load the HTML file and strip all tags except h1, table, tr, th and td. Of course you can also strip off the styles, use your own colors or link directly to the official stylesheet.
CODE
<link href="http://hcc.reclamation.dk/report.css" rel="stylesheet" type="text/css" />
<?php
$filename = basename(@$_GET['uid'] . '.html');
if (file_exists($filename))
{
$text = file_get_contents('/upload_dir/' . $filename);
echo chop(strip_tags($text, '<h1><table><tr><th><td>'));
}
?>
<?php
$filename = basename(@$_GET['uid'] . '.html');
if (file_exists($filename))
{
$text = file_get_contents('/upload_dir/' . $filename);
echo chop(strip_tags($text, '<h1><table><tr><th><td>'));
}
?>
4. Distribution
Distribute your plugin by putting a zipped version on a Website and send the link via email or post it in a forum post, maybe with some instructions. Don't send the plain batch file directly via email as attachment. All major mail servers filter out executable files, even if they are zipped. Please provide a small readme.txt with your plugin, containing system requirements, known issues and a way to contact you.
Please make sure you include the Plugin Folder into the zip! If you use WinZip or 7Zip the correct method is to rightclick on "MyUploader" and select Zip -> Add to "MyUploader.zip".
CODE
MyUploader\export.bat
MyUploader\config.ini
MyUploader\readme.txt
MyUploader\upload.exe
MyUploader\Images\logo.bmp
MyUploader\Images\hello_world.png
MyUploader\config.ini
MyUploader\readme.txt
MyUploader\upload.exe
MyUploader\Images\logo.bmp
MyUploader\Images\hello_world.png
Top